Javascript Es6 Class Static Method !!
javascript - ES6 - Call static method within a class - Stack Overflow
Both of the answers here are correct and good, but I wanted to throw in an added detail based on this question title. When I saw "ES6 - Call static method within a class" it sounded like "call a static method (from a non-static method If you are calling the static function from inside an instance, the right way to refer to the static function of the class is:It's the same as calling a method on an ordinary object. If you call the GeneralHelper.isProd() method, the GeneralHelper will be available as this in the method, so you can use
This will however not work when the method is passed around as a callback function, just as usual. Also, it might be different from accessing GeneralHelper explicitly when someone inherits isProd from your class and overwrites is, InheritedHelper.isProd() will produce other results.
If you're looking to call static methods from instance methods, see here. Also notice that a class which only defines static methods is an oddball, you may want to use a plain object instead.
Both of the answers here are correct and good, but I wanted to throw in an added detail based on this question title.
When I saw "ES6 - Call static method within a class" it sounded like "call a static method (from a non-static method) within a class". Def not what the initial question asker is asking in the details.
But for anyone who wants to know how to call a static method from a non-static method within a class you can do it like this:
The idea is that the static method is can be called without creating a new instance of the class. That means you can call it inside of a instance's method the same way you'd call it outside of the instance.
Again, I know that's not what the detail of the question was asking for, but this could be helpful other people.
site design / logo © 2021 Stack Exchange Inc; user contributions licensed under cc by-sa. rev 2021.7.16.39771
By clicking âAccept all cookiesâ, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy.
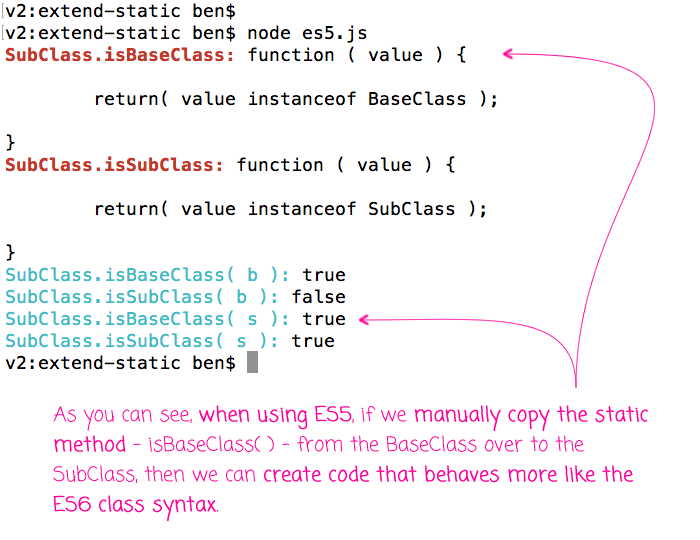
Static Methods in ES6. Static methods, like many other… | by - Medium
Static methods, like many other features introduced in ES6, are meant to provide class-specific methods for object-oriented programming in Javascript. Static methods in Javascript are similar to Static methods, like many other features introduced in ES6, are meant to provide class-specific methods for object-oriented programming in Javascript. Static methods in Javascript are similar to class methods in Ruby. To declare a static method, simply prefix a method declaration with the word static inside the class declaration.As MDN describes it, âStatic methods are called without instantiating their class and are also not callable when the class is instantiated. Static methods are often used to create utility functions for an application.â In other words, static methods have no access to data stored in specific objects.
Since these methods operate on the class instead of instances of the class, they are called on the class. There are two ways to call static methods:
Note that for static methods, the this keyword references the class. You can call a static method from another static method within the same class with this.
With the introduction of classes in ES6, we can now utilize prototype-based inheritance, super calls, constructors, instance and static methods, which make interoperability much easier. Say we have a subclass to a parent class, any static methods that we declared are available to the subclasses as well. This was not available in ES5 unless the methods are explicitly copied over.
Note that static properties are a separate concept from static methods, and are still under development. The rationale for having static properties is best explained here:
Currently itâs possible to express static methods on a class definition, but it is not possible to declaratively express static properties. As a result people generally have to assign static properties on a class after the class declaration â which makes it very easy to miss the assignment as it does not appear as part of the definition.
javascript - Call static methods from regular ES6 class methods - Stack
What's the standard way to call static methods? I can think of using constructor or using the name of the class itself, I don't like the latter since it doesn't feel necessary. Is the former the What's the standard way to call static methods? I can think of using constructor or using the name of the class itself, I don't like the latter since it doesn't feel necessary. Is the former the recommended way, or is there something else?Both ways are viable, but they do different things when it comes to inheritance with an overridden static method. Choose the one whose behavior you expect:
Referring to the static property via the class will be actually static and constantly give the same value. Using this.constructor instead will use dynamic dispatch and refer to the class of the current instance, where the static property might have the inherited value but could also be overridden.
This matches the behavior of Python, where you can choose to refer to static properties either via the class name or the instance self.
If you expect static properties not to be overridden (and always refer to the one of the current class), like in Java, use the explicit reference.
I stumbled over this thread searching for answer to similar case. Basically all answers are found, but it's still hard to extract the essentials from them.
Keep in mind that using this isn't working this way when using arrow functions or invoking methods/getters explicitly bound to custom value.
If you are planning on doing any kind of inheritance, then I would recommend this.constructor. This simple example should illustrate why:
The named class will not deal with inheritance nicely unless you explicitly redefine every function that makes a reference to the named Class. Here is an example:
You can see from this that test4 still thinks it's in the super class; in this example it might not seem like a huge deal, but if you are trying to reference member functions that have been overridden or new member variables, you'll find yourself in trouble.
site design / logo © 2021 Stack Exchange Inc; user contributions licensed under cc by-sa. rev 2021.7.16.39771
By clicking âAccept all cookiesâ, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy.
javascript - ES6 class static method vs function - Stack Overflow
The first one is the traditional approach, i.e. module.exports = random: () => Math.random (), ; And the second option is to use ES6 class with static methods, e.g. class MyMath static random () return Math.random (); module.exports = MyMath; From programming/unit testing's perspective which one is better? Or they are pretty much the same because ES6 class essentially is a syntactic sugar?Update: Thanks for the people who commented. I saw those questions asking class static method v.s. instance method, or prototype methods v.s. object method but mine is more like class static method v.s. Object method.
Using static-only classes as namespaces in JavaScript is the remnant of languages where a class is the only available entity. Modules already act as namespaces.
With the class syntax you might give more than you really intended, it is a constructor that can be invoked with new, while with the object literal syntax you give a non-function object. As that is really what you want to expose, go for that.
Classes with only static methods are the same as a bunch of isolated functions. In this case you probably don't need to use it.As for unit tests it is pretty much the same since classes with only static methods does not differ that much from functions.
site design / logo © 2021 Stack Exchange Inc; user contributions licensed under cc by-sa. rev 2021.7.16.39771
By clicking âAccept all cookiesâ, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy.
ecmascript 6 - ES6 Javascript: Calling static methods from classes with
Arrow functions inherit their this from the outer scope rather than their this depending on their calling context. Since staticMethod2 tries to access this.staticMethod, that will only work if this refers to ClassWithStaticMethod - that is, if staticMethod2 is a standard function, not an arrow function. In the sense, that I can still access to the staticMethod() method, but I am not able to access to the other method within the first method. I get undefined, and if I useArrow functions inherit their this from the outer scope rather than their this depending on their calling context. Since staticMethod2 tries to access this.staticMethod, that will only work if this refers to ClassWithStaticMethod - that is, if staticMethod2 is a standard function, not an arrow function.
You also need to invoke this.staticMethod(). (const yee = this.staticMethod; will result in the staticMethod being coerced to a string, rather than being called)
That's one quirk with arrow functions when it comes to general use: they have generic scoping of this. (That's why we have to use function() if you want a better call stack). In the second method, this refers to the calling context: window.
As mentioned below in the comment, do not use the short-hand syntax for your convenience; there is a reason we have so many options.
site design / logo © 2021 Stack Exchange Inc; user contributions licensed under cc by-sa. rev 2021.7.16.39771
By clicking âAccept all cookiesâ, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy.
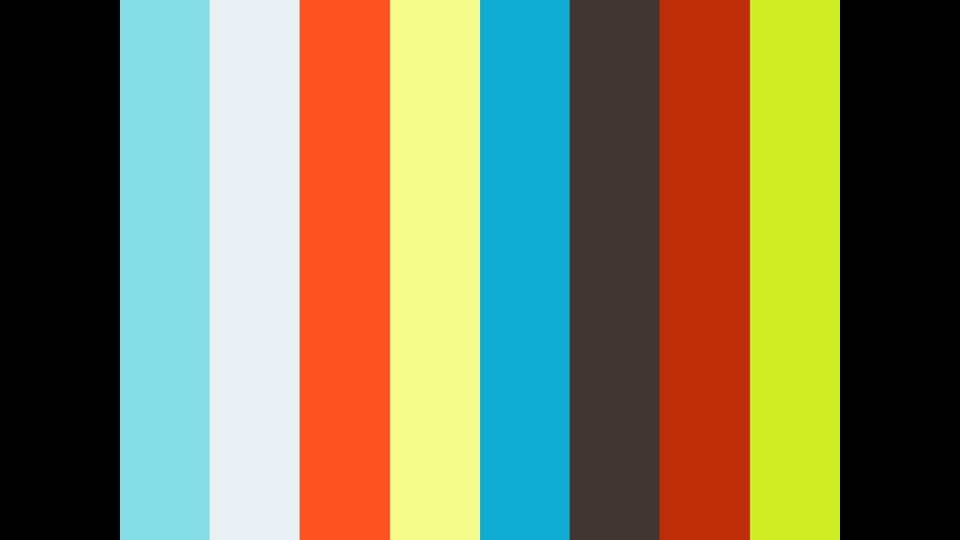
javascript - import class and call static method with es6 modules with
While this code may resolve the OP's issue, it is best to include an explanation as to how your code addresses the OP's issue. In this way, future visitors can learn from your post, and apply it to their own code. I don't have enough reputation to comment, the alexpods's answer is perfect, but for matters of understanding our friend Ced asked:Why do we need the default in the 2nd example ? In other words why can't we have export EmberReflux directly ?
site design / logo © 2021 Stack Exchange Inc; user contributions licensed under cc by-sa. rev 2021.7.16.39771
By clicking âAccept all cookiesâ, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy.
JavaScript Static Method
JavaScript static methods are shared among instances of a class. Thus, they are associated with the class, not any particular instance of that class. The static methods are called via the class name, not the instances of the class. Use the className.staticMethodName () or this.constructor.staticMethodName () to call a static method in a class By definition, static methods are associated with a class, not the instances of that class. Thus, static methods are useful for defining helper or utility methods.The createAnonymous() method is considered a static method because it doesn’t depend on any instance of the Person type for its property values.
If you attempt to call the static method from an instance of the class, you’ll get an error. For example:
To call a static method from a class constructor or an instance method, you use the class name, followed by the . and the static method:
static - JavaScript | MDN
The static keyword defines a static method or property for a class. Neither static methods nor static properties can be called on instances of the class. Instead, they're called on the class itself.미나미 블로그 :: [JavaScript] ES6 Class Static Method
[JavaScript] ES6 Class Static Method . 클래스에 static으로 명시된 함수는 클래스로 호출이 가능하나, 생성된 인스턴스에서는 사용이 불가능하다.Call ES5 class method from static method - Stack Overflow
JavaScript is not based on classes like Java, it is based on prototypes. With ES5 syntax, there are two ways to create what you call a "class": An object literal. A constructor function. When you use an object literal, your class looks like this: var myClass = myAttribute: "foo", myMethod: function () return "bar"; ; javascript es6 class static methodjavascript es6 class static method
javascript adalah,javascript array,javascript alert,javascript array push,javascript array length,javascript add class,javascript array filter,javascript array to string,javascript async await,javascript append,es6 adalah,es6 arrow function,es6 array,es6 array methods,es6 array filter,es6 array sort,es6 array map,es6 array find,es6 async await,es6 array contains,class action adalah,class action,class adalah,class artinya,class action lawsuit,class act,class a amplifier,class act meaning,class a ip address,class abstract adalah,static adalah,static analysis,static apnea,static artinya,static and dynamic,static antonym,static and ben el,static animal crossing,static and dynamic characters,static application security testing,method adalah,method acting,method artinya,method acting adalah,method array di php adalah,method actors,method acting meaning,method abstract adalah,method and methodology,method actor adalah
Posting Komentar untuk "Javascript Es6 Class Static Method !!"