Javascript Es6 Class Syntax !!
JavaScript ES6 Class Syntax - Cory Rylan
ES6 brings exciting features to JavaScript including new syntax improvements. This post I am going to cover the new Class syntax. JavaScript has been a prototypal based language using object prototypes to create object inheritance and code reuse. The new ES6 Class adds a new syntax on top of traditional prototypes. My name is Cory Rylan. Google Developer Expert, speaker, and Staff UI Engineer at VMware Clarity Design System.ECMAScript 2015 or more well known as ES6 is the next specification for JavaScript. ES6 brings exciting features to JavaScript including new syntax improvements. This post I am going to cover the new Class syntax. JavaScript has been a prototypal based language using object prototypes to create object inheritance and code reuse. The new ES6 Class adds a new syntax on top of traditional prototypes.
Something I cannot stress enough is the new Class is syntactic sugar on prototypes. Under the hood, ES6 Classes are still using prototypal inheritance. If you are unfamiliar with prototypes I would suggest you read my previous post on JavaScript Prototypal Inheritance.
In ES5 or the current widely supported version of JavaScript, we use prototypes to create object inheritance. Before ES6, we used function constructors similar to this.
ES2015/ES6 has the new reserved keyword Class with a constructor statement. So the ES2015/ES6 equivalent of our Person function constructor would be the following.
The new syntax gives us a dedicated constructor statement that runs on objectcreation. Constructors are helpful for any object initialization logic.
ES6 classes brings a new syntax for getters and setters on object properties Get and set allows us to run code on the reading or writing of a property. ES5 had getters and setters as well but was not widely used because of older IE browsers. ES5 getters and setters did not have as nice of a syntax that ES6 brings us. So let's create a get and set for our name property.
In our class above we have a getter and setter for our name property. We use _ convention to create a backing field to store our name property. Without this every time get or set is called it would cause a stack overflow. The get would be called and which would cause the get to be called again over and over creating an infinite loop.
Something to note is that our backing field this._name is not private. Someone could still access bob._name and retrieve the property. To achieve private state on objects, you would use ES6 symbol and module to create true encapsulation and private state. Private methods can be created using module or traditional closures using an IIFE. Using languages like TypeScript you can get compile-time enforcement of private properties and methods.
Now let's look into inheritance using traditional prototypes in ES5 syntax. We will create a Programmer object to inherit our Person object. Our programmer object will inherit person and also have a writeCode() method.
You can see the class syntax offers a clean syntax for prototypal inheritance. One detail you may notice is the super() keyword. The super keyword lets us call the parent object that is being inherited. It is good advice to avoid this as this can cause an even tighter coupling between your objects, but there are occasions where it is appropriate to use. In this case, it can be used in the constructor to assign to the super constructor. If the Person constructor contained any logic, custom getters or setters for the name property we would want to use the super and not duplicate the logic in the Programmer class. If a constructor is not defined on a child class the super class constructor will be invoked by default.
Here is one final look at our Person and Programmer classes. The getters and setters are not necessary in this use case but are there to demonstrate the new syntax.
A codepen.io demo of the code above can be found here. ES6 classes bring some syntactical sugar to prototypes.Just remember that is all ES6 classes are, syntactic sugar. Remember classes are just one of many options to organize and structure code. There are many other great design patterns for code reuse such as the module pattern.
ES6 brings some great improvements to making JavaScript a more productive programming language and is already being implemented in browsers today. To start writing ES6 today check out Babel JS (formerly 6to5) a transpiler that transpile ES6 JavaScript to ES5.
Save development time, improve product consistency and ship everywhere. With this new Course and E-Book learn how to build UI components that work in any JavaScript framework such as Angular, Vue, React, and more!
Learn a brief overview on Web Components and the latest tech available to build and distribute components across the Web.
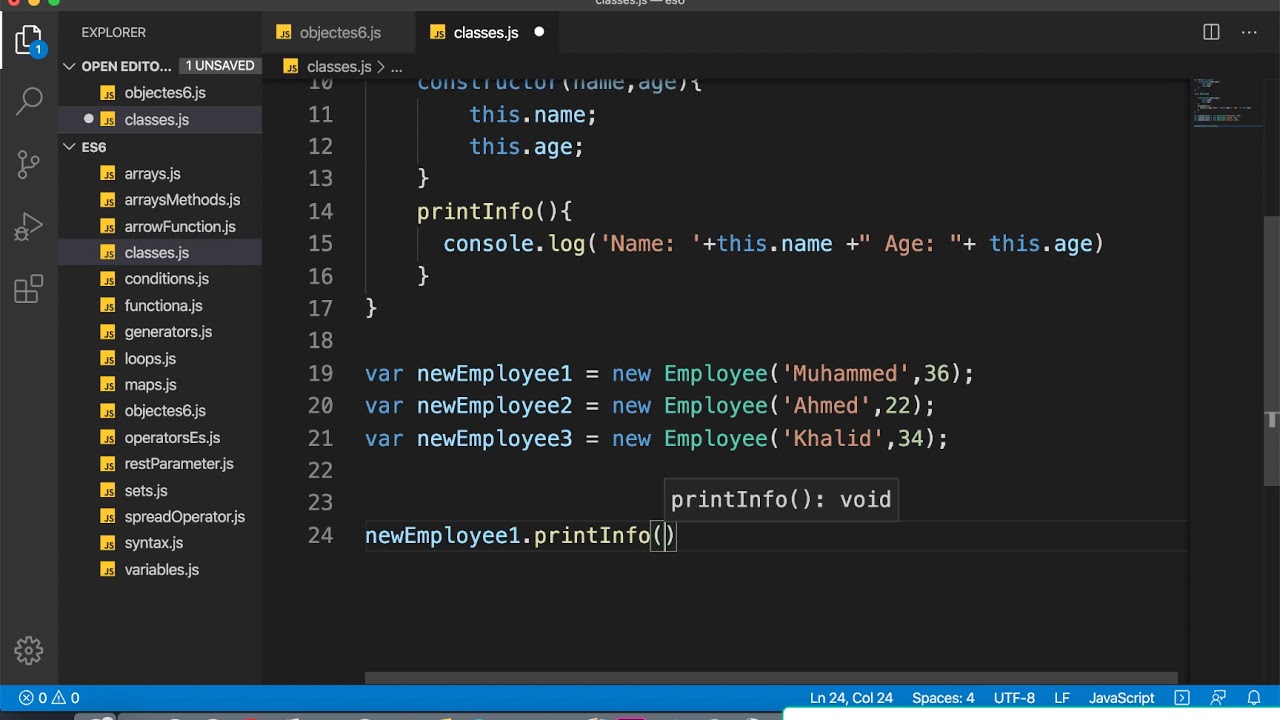
JavaScript ES6: Classes. Objects in programming languages… | by Luke
JavaScript ES6: Classes. Objects in programming languages provide us with an easy way to model data. Let’s say we have an object called user. The user object has properties: values that contain Objects in programming languages provide us with an easy way to model data. Let’s say we have an object called user. The user object has properties: values that contain data about the user, and methods: functions that define actions that the user can perform. This focus on “objects†and “actions†is the basis of Object Oriented Programming.OOP describes a way to write programs. This way focuses on data: stored as object properties, and actions: stored as object methods. The notion behind this way of writing code is that it more closely reflects how we think in reality. In OOP, everything is an object, and any actions we need to perform on data (logic, modifications, e.c.t) are written as methods of an object.
Going back to the user object, even in the smallest real world application, we’re going to have multiple user objects. If we had to hard code each one, we may as well go back to pen and paper:
Since each object has the same type of properties, it would be better to have a template for the user objects that we just populate with data when we want to create a new user object:
Now we have a template for each user object. The User function is an example of a constructor. A constructor is a function that is called each time an object is created (also referred to as instantiated). The User constructor creates the properties of the object (this.name, this.age, this.email) and assigns them the value of the parameters passed to it (name, age, email).
This way of writing Object Oriented JavaScript works. But as with other things, ES6 gives us a better way of doing it.
In ES6 we can create classes. If you’ve come from a language like PHP or Python, this will be familiar to you. Let’s take a look at the syntax:
The class function basically creates a template that we can use to create objects later. The constructor() method is a special method called when an instance of the User class is created. This is essentially the same as the User function we defined in the pre-ES6 example.
Classes can also contain static methods. A static method is a function that is bound to the class, not an object. A static method cannot be called from an instance of the class. Here’s an example:
Let’s take a quick look at getters and setters. One of the core concepts of OOP is encapsulation. An important part of encapsulation is that data (object properties) should not be directly accessed or modified from outside the object. To access or modify a property we would use a getter (access) or a setter (modify), which are specific methods we define in our class. Let’s take a look at a quick example:
Currently there is no native support for private properties in JavaScript (it is possible to mimic private properties but we’re not going to go into that). So all the properties we’ve declared can be directly accessed from outside the object. But following encapsulation is best practice for OOP.
Inheritance: Classes can also inherit from other classes. The class being inherited from is called the parent, and the class inheriting from the parent is called the child. In our example, another class, let’s say Administrator, can inherit the properties and methods of the User class:
In the above example, User is the parent and Administrator is the child. There’s a few important things to note. Firstly, when we create the child class we need to state that it extends the parent class. Then we need to pass whatever properties we want to inherit from the parent to the child’s constructor, as well as any new properties that we will define in the child class. Next, we call the super method. Notice that we pass it the values that we pass the child class when creating the sara object. These values are defined in the parent’s constructor so we need to run it in order for the values to be instantiated. Now we can define our child class’s properties and methods.
This has been a brief introduction to OOP in JavaScript with ES6 class syntax. OOP is a massive subject, but hopefully this article has shown you the benefits of using it when writing code. ES6’s class syntax gives us an easier way to work with objects and inheritance, and makes writing Object Oriented JavaScript a breeze.
javascript - What benefits does ES2015 (ES6) `class` syntax provide
What benefits does ES2015 (ES6) class syntax provide? Briefly: If you don't use constructor functions in the first place, preferring Object.create or similar, class isn't useful to you. If you do use constructor functions, there are some benefits to class: The syntax is simpler and less error-prone. What's the benefit of using class syntax? I read that public/private/static will be part of ES7, is that a reason?Moreover, is class a different kind of OOP or it still JavaScript's prototypical inheritance? Can I modify it using .prototype ? Or is it just the same object but two different ways to declare it.
The new class syntax is, for now, mostly syntactic sugar. (But, you know, the good kind of sugar.) There's nothing in ES2015-ES2020 that class can do that you can't do with constructor functions and Reflect.construct (including subclassing Error and Array¹). (It is likely there will be some things in ES2021 that you can do with class that you can't do otherwise: private fields, private methods, and static fields/private static methods.)
It's the same prototypical inheritance we've always had, just with cleaner and more convenient syntax if you like using constructor functions (new Foo, etc.). (Particularly in the case of deriving from Array or Error, which you couldn't do in ES5 and earlier. You can now with Reflect.construct [spec, MDN], but not with the old ES5-style.)
Yes, you can still modify the prototype object on the class's constructor once you've created the class. E.g., this is perfectly legal:
By providing a specific idiom for this, I suppose it's possible that the engine may be able to do a better job optimizing. But they're awfully good at optimizing already, I wouldn't expect a significant difference.
Briefly: If you don't use constructor functions in the first place, preferring Object.create or similar, class isn't useful to you.
It's much easier (and again, less error-prone) to set up inheritance hierarchies using the new syntax than with the old.
class defends you from the common error of failing to use new with the constructor function (by having the constructor throw an exception if this isn't a valid object for the constructor).
Calling the parent prototype's version of a method is much simpler with the new syntax than the old (super.method() instead of ParentConstructor.prototype.method.call(this) or Object.getPrototypeOf(Object.getPrototypeOf(this)).method.call(this)).
As you can see, lots of repeated and verbose stuff there which is easy to get wrong and boring to retype (which is why I wrote a script to do it, back in the day).
¹ "There's nothing in ES2015-ES2018 that class can do that you can't do with constructor functions and Reflect.construct (including subclassing Error and Array)"
ES6 classes are syntactic sugar for the prototypical class system we use today. They make your code more concise and self-documenting, which is reason enough to use them (in my opinion).
The second version isn't much more complicated, it is more code to maintain. When you get inheritance involved, those patterns become even more complicated.
Because the classes compile down to the same prototypical patterns we've been using, you can do the same prototype manipulation on them. That includes adding methods and the like at runtime, accessing methods on Foo.prototype.getBar, etc.
There is some basic support for privacy in ES6 today, although it's based on not exporting the objects you don't want accessible. For example, you can:
A lot of libraries have tried to support or solve this, like Backbone with their extends helper that takes an unvalidated hash of method-like functions and properties, but there's no consist system for exposing prototypical inheritance that doesn't involve mucking around with the prototype.
As JS code becomes more complicated and codebases larger, we've started to evolve a lot of patterns to handle things like inheritance and modules. The IIFE used to create a private scope for modules has a lot of braces and parens; missing one of those can result in a valid script that does something entirely different (skipping the semicolon after a module can pass the next module to it as a parameter, which is rarely good).
site design / logo © 2021 Stack Exchange Inc; user contributions licensed under cc by-sa. rev 2021.7.16.39771
By clicking “Accept all cookiesâ€, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy.
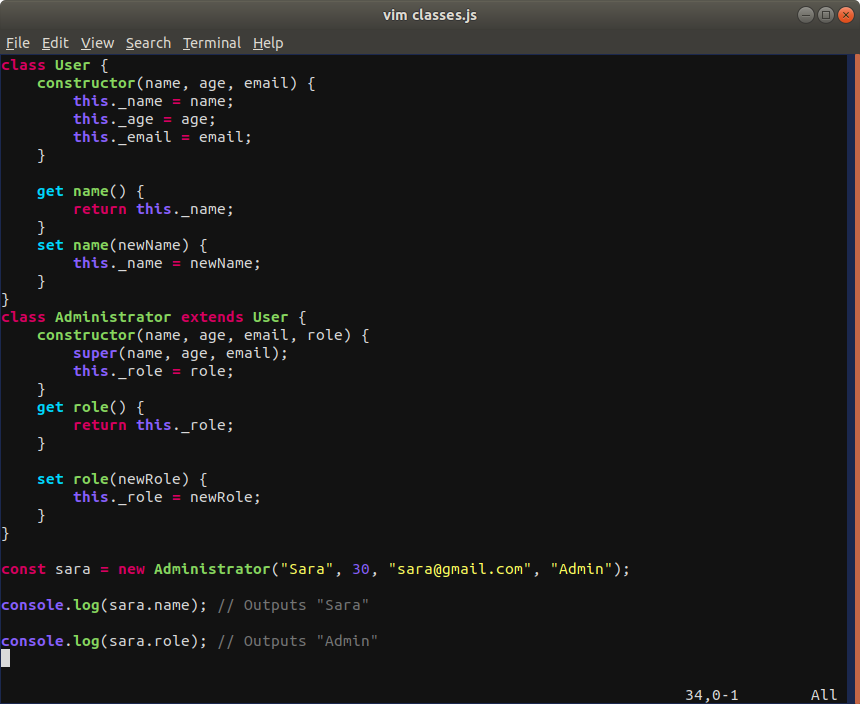
JavaScript Class Fundamentals: Introduction to ES6 Class
ES6 class declaration. ES6 introduced a new syntax for declaring a class as shown in this example: class Person constructor (name) this .name = name; getName () return this .name; Code language: JavaScript (javascript) This Person class behaves like the Person type in the previous example.Legit JavaScript Classes > JavaScript for PHP Geeks: ES6/ES2015 (New
You can also remove the semicolon after the method, just like in PHP: Moving everything else into the new class syntax is easy: remove $.extend (helper.prototype) and move all of the methods inside of the class: And congratulations! We just created a new ES2015 class.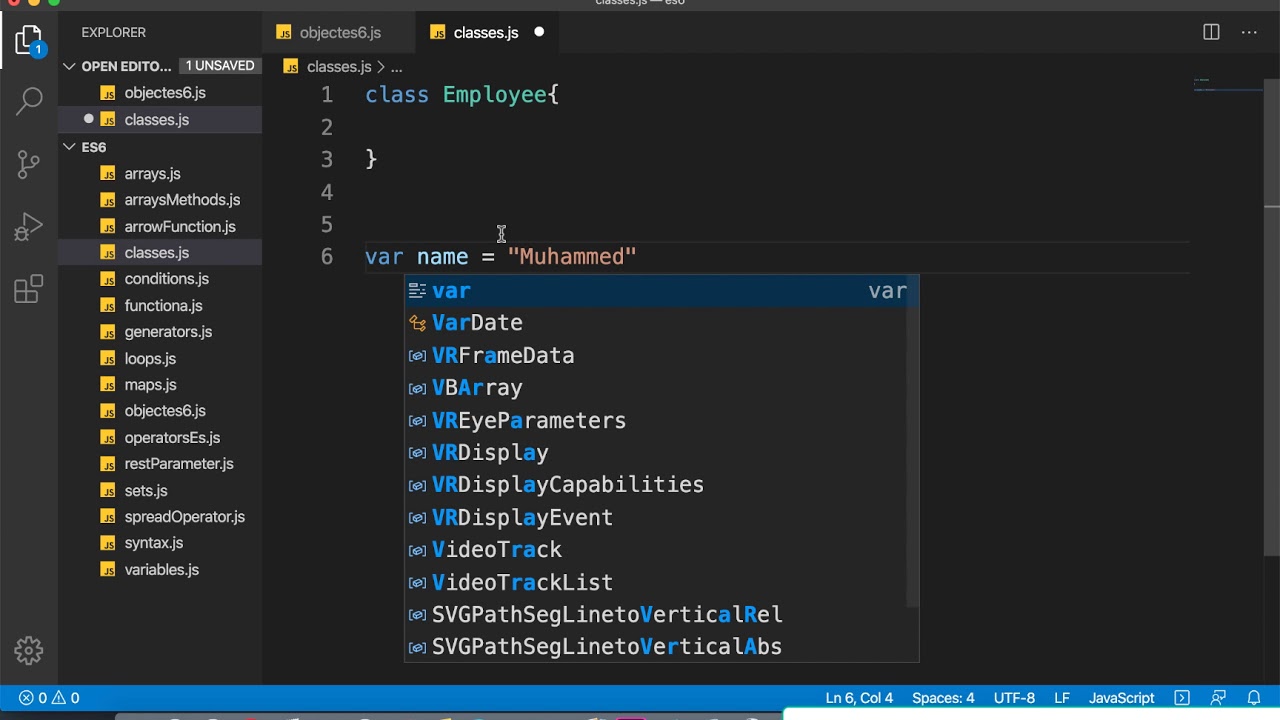
LAB: ES6 Classes | code-401-javascript-guide
Implement both Car and Motorcycle as Vehicles using only Javascript ES6 Class syntax Implementation Details When running this REPL, you will see that there is some output that will show you that a Car and Motorcycle can be created properly using a Constructor Function and Prototype methods.JavaScript ES6 - W3Schools
The For/Of Loop. The JavaScript for/of statement loops through the values of an iterable objects. for/of lets you loop over data structures that are iterable such as Arrays, Strings, Maps, NodeLists, and more. The for/of loop has the following syntax: for ( variable of iterable) {. // code block to be executed.Classes - JavaScript | MDN
Classes are a template for creating objects. They encapsulate data with code to work on that data. Classes in JS are built on prototypes but also have some syntax and semantics that are not shared with ES5 class-like semantics.Penjelasan dari getter dan setter di Class Javascript - Kotakode
Jadi di ES6 Javascript, telah di [perkenalkan](https://coryrylan.com/blog/javascript-es6-class-syntax) sintaks baru untuk `Class` dengan adanya `getter` dan `setter`. ### Getter: 1. Mengambil data dari property asli lalu di `return`. 2. Atau bisa juga sebelum di `return`, datanya bisa di olah terlebih dahulu.Class basic syntax - JavaScript
In object-oriented programming, a class is an extensible program-code-template for creating objects, providing initial values for state (member variables) and implementations of behavior (member functions or methods). javascript es6 class syntaxjavascript es6 class syntax
javascript adalah,javascript array,javascript alert,javascript array push,javascript array length,javascript add class,javascript array filter,javascript array to string,javascript async await,javascript append,es6 adalah,es6 arrow function,es6 array,es6 array methods,es6 array filter,es6 array sort,es6 array map,es6 array find,es6 async await,es6 array contains,class action adalah,class action,class adalah,class artinya,class action lawsuit,class act,class a amplifier,class act meaning,class a ip address,class abstract adalah,syntax adalah,syntax analysis,syntax artinya,syntax and grammar,syntax and morphology,syntax analyzer,syntax and semantics,syntax advisor,syntax admiration,syntax arduino
Posting Komentar untuk "Javascript Es6 Class Syntax !!"