Js Class Singleton Es6 !!
javascript - Converting Singleton JS objects to use ES6 classes - Stack
@dejakob Referring to this in a static method will reference the CLASS (prototype, to be exact), not the object. By using a symbol which is defined out of the class, we are essentially creating a private class member (which is different from a private instance member). I'd argue that singletons (classes that manage their own singleton lifetime) are unnecessary in any language. That is not to say that singleton lifetime is not useful, just that I prefer that something other than the class manage the lifetime of an object, like a DI container.That being said, the singleton pattern CAN be applied to JavaScript classes, borrowing the "SingletonEnforcer" pattern that was used in ActionScript. I can see wanting to do something like this when porting an existing code base that uses singletons into ES6.
In this case, the idea is that you make a private (via an un exposed Symbol) static singleton instance, with a public static instance getter. You then restrict the constructor to something that has access to a special singletonEnforcer symbol that is not exposed outside of the module. That way, the constructor fails if anyone other than the singleton tries to "new" it up. It would look something like this:
If you really want to use a class in your singleton approach, I would recommend against using "static" as it more confusing than good for a singleton at least for JS and instead return the instance of the class as a singleton like so
This way you can still use all the class things you like that JavaScript offers but it will reduce the confusion as IMO static isn't fully supported in JavaScript classes anyway as it is in typed languages such as c# or Java as it only supports static methods unless you just fake it and attach them directly to a class (at the time of this writing).
I had to do the same so here is a simple and direct way of doing a singleton, curtsy to singleton-classes-in-es6
site design / logo © 2021 Stack Exchange Inc; user contributions licensed under cc by-sa. rev 2021.7.16.39771
By clicking “Accept all cookiesâ€, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy.

Singletons in ES6 - The Good, The Bad, The Ugly - Qvault
A singleton is a class that allows only a single instance of itself to be created and gives access to that created instance. It contains static variables that can accommodate unique and private instances of itself. We use it in scenarios when a user wants to restrict instantiation of a class to only one object. Singletons are fairly controversial as far as I can tell, especially in JavaScript programming. Let’s take a look at what they are, when to (maybe) use them, and when not to.A singleton is a class that allows only a single instance of itself to be created and gives access to that created instance. It contains static variables that can accommodate unique and private instances of itself. We use it in scenarios when a user wants to restrict instantiation of a class to only one object. This is helpful usually when a single object is required to coordinate actions across a system.
Usually, in object-oriented programming, the idea is to define classes and create multiple instances of that class, each with their own state. This keeps code DRY and easy to maintain.
For the purpose of this article, we will assume we are using ES6 modules in our front-end React or Vue project. An example of a singleton we might want could be:
As stated earlier, a singleton is dangerously close to being a global variable, and we don’t like those. There is one important difference:
The singleton instance isn’t actually globally scoped: in order to modify state the caller must import the class and use getters/setters. This makes access more explicit and controlled. Not only are the ways in which the state can be modified explicitly defined, but files that use the state must ‘import’ it.
The idea is to use the simplest, most-controlled solution we reasonably can. In order of least evil –> most evil:
Redux, Vuex, singletons, and global variables all suck. Try not to use them. I’m just trying to point out that they all suck to varying degrees. Good luck.
Follow and hit me up on Twitter @q_vault if you have any questions or comments. If I’ve made a mistake in the article, please let me know so I can get it corrected!
javascript - ES6 Singleton vs Instantiating a Class once - Stack Overflow
I see patterns which make use of a singleton pattern using ES6 classes and I am wondering why I would use them as opposed to just instantiating the class at the bottom of the file and exporting the instance. I see patterns which make use of a singleton pattern using ES6 classes and I am wondering why I would use them as opposed to just instantiating the class at the bottom of the file and exporting the instance. Is there some kind of negative drawback to doing this? For example:What is the difference from using the following Singleton pattern? Are there any reasons for using one from the other? Im actually more curious to know if the first example I gave can have issues that I am not aware of.
I would recommend neither. This is totally overcomplicated. If you only need one object, do not use the class syntax! Just go for
and then importing like as below in multiple files would point to same instance and a way of creating Singleton pattern.
Whereas the other way of exporting the class directly is not singleton as in the file where we are importing we need to new up the class and this will create a separate instance for each newing up Exporting class only:
Another reason to use Singleton Pattern is in some frameworks (like Polymer 1.0) you can't use export syntax.That's why second option (Singleton pattern) is more useful, for me.
site design / logo © 2021 Stack Exchange Inc; user contributions licensed under cc by-sa. rev 2021.7.16.39771
By clicking “Accept all cookiesâ€, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy.
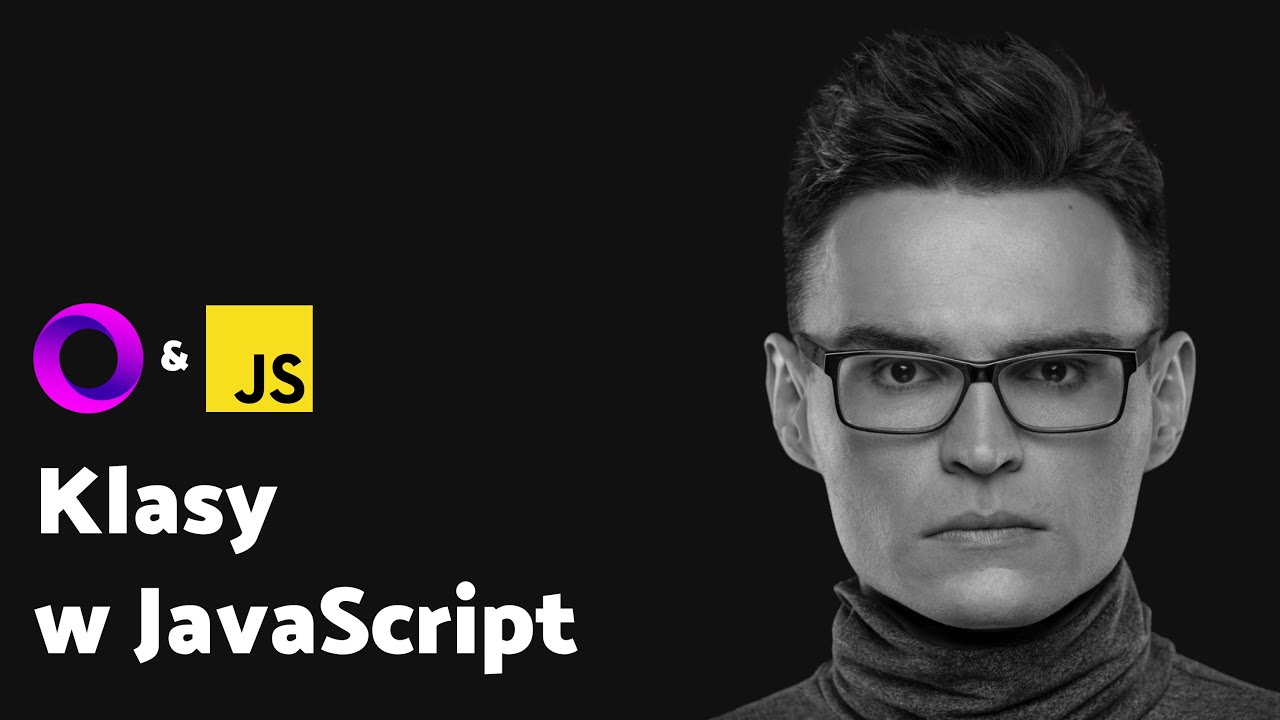
Singleton Pattern in ES6 and ES7 : Adam Bien's Weblog
Accessing AWS Systems Manager / Parameter Store's Configuration from Quarkus A Soldering, Agile, Geek Lawyer using Java and Quarkus--an airhacks.fm podcast Serverless, RESTful Searches, Jakarta EE Servers, AWS, Continuous Testing, Siemens Airhook and Continuous Testing with Quarkus--or 88th airhacks.tv J4K 2021: The Future is Now: Overview of Next Generation Cloud Java Runtimes Clouds The code above uses ECMAScript 2015/ES6 (classes) and ECMAScript 2016/ES7 (static fields) features.It transcompiled, "old school" ES5 representation looks like:See you at Web, MicroProfile and Java EE Workshops at Munich Airport, Terminal 2 or Virtual Dedicated Workshops / consulting. Is Munich's airport too far? Learn from home: airhacks.io.
NEW live, virtual workshop: Building Serverless Java Apps on AWS Cloud December 16th, 2021 and Testing, Monitoring, Resiliency December 9th, 2021 are open for registration. Also via: meetup.com/airhacks and airhacks.eventbrite.com
Posted by Sebastian Cheung on September 13, 2016 at 11:15 PM CEST #
Posted by Ahmed Khaled on March 14, 2020 at 11:31 AM CET #
Amazingly, strings are a problem throughout so many languages, C, C++, Java, Python How could something so simple as a bunch of characters repr[ ]
"It is recommended to obtain a Path via the Path.of methods instead of via the get methods defined in this class as this class may be depreca[ ]
can you give an example of how to test click a radio button and also clicking the submit button on the form?
very interesting video. My question is how do you deploy such application? Do you have a WAR with react js application in WEB-INF or do you run it[ ]
JavaScript Design Patterns: The Singleton - SitePoint
RRP $11.95. Get the book free! In this article, we’ll dig into the best way to implement a singleton in JavaScript, looking at how this has evolved with the rise of ES6. Among languages used in In this article, we’ll dig into the best way to implement a singleton in JavaScript, looking at how this has evolved with the rise of ES6.Among languages used in widespread production, JavaScript is by far the most quickly evolving, looking less like its earliest iterations and more like Python, with every new spec put forth by ECMA International. While the changes have their fair share of detractors, the new JavaScript does succeed in making code easier to read and reason about, easier to write in a way that adheres to software engineering best practices (particularly the concepts of modularity and SOLID principles), and easier to assemble into canonical software design patterns.
ES6 (aka ES2015) was the first major update to the language since ES5 was standardized in 2009. Almost all modern browsers support ES6. However, if you need to accommodate older browsers, ES6 code can easily be transpiled into ES5 using a tool such as Babel. ES6 gives JavaScript a ton of new features, including a superior syntax for classes, and new keywords for variable declarations. You can learn more about it by perusing SitePoint articles on the subject.
In case you’re unfamiliar with the singleton pattern, it is, at its core, a design pattern that restricts the instantiation of a class to one object. Usually, the goal is to manage global application state. Some examples I’ve seen or written myself include using a singleton as the source of config settings for a web app, on the client side for anything initiated with an API key (you usually don’t want to risk sending multiple analytics tracking calls, for example), and to store data in memory in a client-side web application (e.g. stores in Flux).
A singleton should be immutable by the consuming code, and there should be no danger of instantiating more than one of them.
Note: there are scenarios when singletons might be bad, and arguments that they are, in fact, always bad. For that discussion, you can check out this helpful article on the subject.
The old way of writing a singleton in JavaScript involves leveraging closures and immediately invoked function expressions . Here’s how we might write a (very simple) store for a hypothetical Flux implementation the old way:
When that code is interpreted, UserStore will be set to the result of that immediately invoked function — an object that exposes two functions, but that does not grant direct access to the collection of data.
However, this code is more verbose than it needs to be, and also doesn’t give us the immutability we desire when making use of singletons. Code executed later could modify either one of the exposed functions, or even redefine UserStore altogether. Moreover, the modifying/offending code could be anywhere! If we got bugs as a result of unexpected modification of UsersStore, tracking them down in a larger project could prove very frustrating.
There are more advanced moves you could pull to mitigate some of these downsides, as specified in this article by Ben Cherry. (His goal is to create modules, which just happen to be singletons, but the pattern is the same.) But those add unneeded complexity to the code, while still failing to get us exactly what we want.
By leveraging ES6 features, mainly modules and the new const variable declaration, we can write singletons in ways that are not only more concise, but which better meet our requirements.
As you can see, this way offers an improvement in readability. But where it really shines is in the constraint imposed upon code that consumes our little singleton module here: the consuming code cannot reassign UserStore because of the const keyword. And as a result of our use of Object.freeze, its methods cannot be changed, nor can new methods or properties be added to it. Furthermore, because we’re taking advantage of ES6 modules, we know exactly where UserStore is used.
Now, here we’ve made UserStore an object literal. Most of the time, going with an object literal is the most readable and concise option. However, there are times when you might want to exploit the benefits of going with a traditional class. For example, stores in Flux will all have a lot of the same base functionality. Leveraging traditional object-oriented inheritance is one way to get that repetitive functionality while keeping your code DRY.
This way is slightly more verbose than using an object literal, and our example is so simple that we don’t really see any benefits from using a class (though it will come in handy in the final example).
One benefit to the class route that might not be obvious is that, if this is your front-end code, and your back end is written in C# or Java, you can employ a lot of the same design patterns in your client-side application as you do on the back end, and increase your team’s efficiency (if you’re small and people are working full-stack). Sounds soft and hard to measure, but I’ve experienced it firsthand working on a C# application with a React front end, and the benefit is real.
It should be noted that, technically, the immutability and non-overridability of the singleton using both of these patterns can be subverted by the motivated provocateur. An object literal can be copied, even if it itself is const, by using Object.assign. And when we export an instance of a class, though we aren’t directly exposing the class itself to the consuming code, the constructor of any instance is available in JavaScript and can be invoked to create new instances. Obviously, though, that all takes at least a little bit of effort, and hopefully your fellow devs aren’t so insistent on violating the singleton pattern.
But let’s say you wanted to be extra sure that nobody messed with the singleness of your singleton, and you also wanted it to match the implementation of singletons in the object-oriented world even more closely. Here’s something you could do:
By adding the extra step of holding a reference to the instance, we can check whether or not we’ve already instantiated a UserStore, and if we have, we won’t create a new one. As you can see, this also makes good use of the fact that we’ve made UserStore a class.
There are no doubt plenty of developers who have been using the old singleton/module pattern in JavaScript for a number of years, and who find it works quite well for them. Nevertheless, because finding better ways to do things is so central to the ethos of being a developer, hopefully we see cleaner and easier-to-reason-about patterns like this one gaining more and more traction. Especially once it becomes easier and more commonplace to utilize ES6+ features.
This is a pattern I’ve employed in production to build the stores in a custom Flux implementation (stores which had a little more going on than our examples here), and it worked well. But if you can see holes in it, please let me know. Also please advocate for whichever of the new patterns you prefer, and whether or not you think object literals are the way to go, or if you prefer classes!
Samier Saeed is a freelance software developer based in Los Angeles. He works in Python, JavaScript, and C#. He is reachable via his website.
javascript - es6 - js class singleton - 入門サンプル
使用法:. Singleton Patternを使用する別の理由は、( Polymer 1.0 ような)いくつかのフレームワークでは、 export 構文を使用できません。. だから、第二の選択肢(シングルトンパターン)が私にとってより有用なのです。. それが役に立てば幸い。. 両方とも同じfunction - Simplest/cleanest way to implement a singleton in JavaScript
@Victor – This is not strange to look for a "singleton pattern" in such language. many Object-oriented languages utilize global variables and still singletons are in use.Create Singleton pattern using ES6 · GitHub
Create Singleton pattern using ES6. GitHub Gist: instantly share code, notes, and snippets.ES6 singleton pattern: constructor return an instance scoped to the
ES6 singleton pattern: constructor return an instance scoped to the module. Raw. SingletonModuleScopedInstance.js. // http://amanvirk.me/singleton-classes-in-es6/. let instance = null; class SingletonModuleScopedInstance {. constructor() {. if (! instance) {.How To Work With Singletons in JavaScript | DigitalOcean
Introduction. The singleton is one of the most well-known design patterns. There are times when you need to have only one instance of a class and no more than that. This one class could be some kind of resource manager or some global lookup for values. js class singleton es6node js singleton class es6
js class singleton es6
js array,js ashigara,js array filter,js alert,js akebono,js array push,js array map,js array length,js array find,js add class,class action adalah,class action,class adalah,class artinya,class action lawsuit,class act,class a amplifier,class act meaning,class a ip address,class abstract adalah,singleton adalah,singleton alcohol percentage,singleton android,singleton anti pattern,singleton android studio,singleton associates pa,singleton argus,singleton accommodation,singleton army base,singleton australia,es6 adalah,es6 arrow function,es6 array,es6 array methods,es6 array filter,es6 array sort,es6 array map,es6 array find,es6 async await,es6 array contains
Posting Komentar untuk "Js Class Singleton Es6 !!"